Embed a Vimeo Video into a React Website
How do you embed a Vimeo video into a React website or application?
In this article, we're going to walk through the process of embedding a Vimeo video into a React application/website. To do most of the heavy lifting, we'll use the ReactPlayer NPM package and React component to embed the video.
Let's get started!
Before working through this article, we assume that you already have a React application created. If you need help creating one, we have an article that lays out 6 different ways to create a React application.
Table Of Contents
Install ReactPlayer NPM Package
First, we need to install the ReactPlayer NPM package. You can install it using one of the below commands:
NPM:
npm install react-player --save
Yarn:
yarn add react-player
Now we're ready to embed the Vimeo video player into your React application.
Use ReactPlayer To Embed A Vimeo Video
Open the page in your React application you want to add the Vimeo media player to and import the react-player
NPM package into the page:
import ReactPlayer from "react-player"
This will make the <ReactPlayer />
component available for use in the page.
Here is how you'd put the component to use:
import React from "react"
import ReactPlayer from "react-player"
function App() {
return (
<div>
<ReactPlayer
url="https://vimeo.com/3155182"
/>
</div>
)
}
export default App
This code will embed a Vimeo video player into your page with the video located at the given url
props value. We also supplied the component with a controls
props value. This tells ReactPlayer
to show the player controls native to Vimeo.
After you save the file and reload the page, you should see the media player in the page.
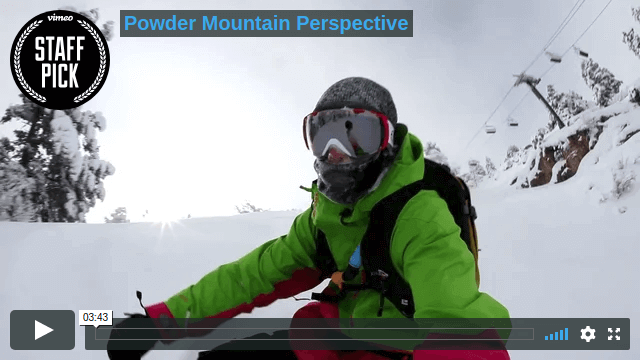
The ReactPlayer
component is now embedded in your webpage!
There are several other props data you can add to the component beyond the url
and control
values. Check out the project's documentation or play around with their demo for more information.