How To Add A Meta Description Tag To A Next.js Website
The meta description is an HTML attribute that gives a brief summary of a web page. Search engines (Google, DuckDuckGo, etc.) often display the meta description value in search results, which can increase the number of people who click-through to your page.
The meta description attribute should be placed inside the head section of the page. And it's best to keep meta descriptions to less than 160 characters in length.
An example of a meta description in a Google search result:
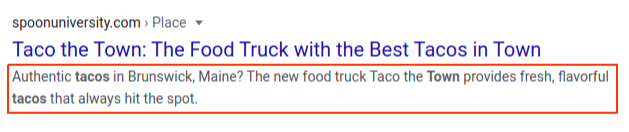
In HTML form, it looks like this:
<head>
<meta name="description" content="An example of a meta description. These show up in search engine results.">
</head>
So, how do you add a meta description attribute to a Next.js website?
Next.js has a built-in Head component for appending elements to the head section of a page.
First, you need to import the next/head
component at the top of your Next.js page:
import Head from "next/head"
Since the next/head
component is included with Next.js, you don't need to install any NPM dependencies to your project.
Then, you can create a new <Head>
component that looks like this:
<Head>
<meta name="description" content="Meta description content goes here." />
</Head>
The component will also look like this when placed in a Next.js page:
import { Component } from "react"
import Head from "next/head"
export default class extends Component {
render() {
return (
<>
<Head>
<meta name="description" content="Meta description content goes here." />
</Head>
<div>Page Content</div>
</>
)
}
}
Notice that the <Head>
component is the first item inside the return()
method. This is to ensure that the tags inside the <Head>
component are picked up properly on client-side navigations.
Save your file and view the page in your favorite browser.
If you open the Developer Tools and examine the head
section of the HTML for your page, you should see the meta description you added.
There you have it! That's how you add a meta description attribute to your Next.js website.
Thanks for reading and happy coding!